Scheduling Future Tasks
Scheduling Future Tasks
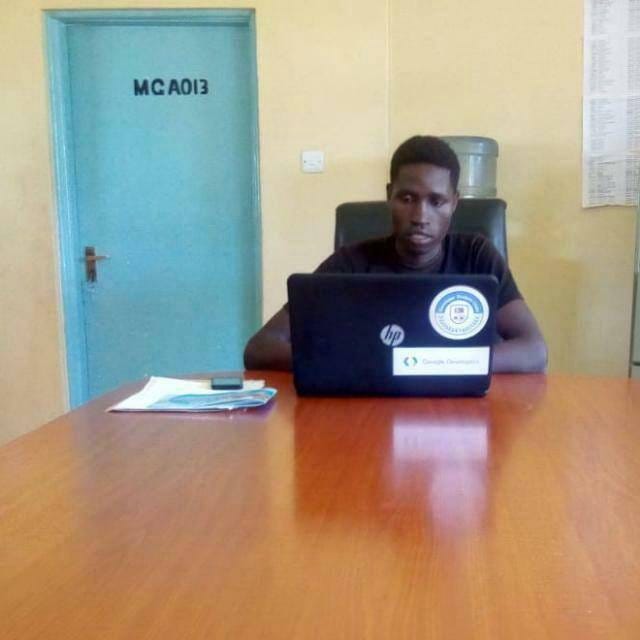
Recently , I was tasked with this project that got me diving into the books sourcing for solutions. In this assignment I was required to build an application that will allow users to schedule various tasks from their app (android) to run particular tasks on an IOT device (remotely).As an Android Engineer my first thoughts went to Work Manager from google’s jetpack APIs. Why was this a bad decision??
Why Work manager will not fit our condition
WorkManager is an API that makes it easy to schedule deferrable, asynchronous tasks that are expected to run even if the app exits or the device restarts. The WorkManager API is a suitable and recommended replacement for all previous Android background scheduling APIs, including FirebaseJobDispatcher, GcmNetworkManager, and Job Scheduler.
- We want the tasks to run even if the mobile app is not online
- In case of a forced stop , the scheduled tasks should still run as earlier expected.
- Once tasks are scheduled , they should run even if the user uninstalls our app.
Server Side Handling
Due to the mentioned constraints I opted for a server side fix, keeping in mind a few constraints.
- The server might be in a different time Zone.
- The server might not be running when the task is to be triggered.
- Result might not be sent back to the server after performing the scheduled task.
Springboot And Job Scheduling
To schedule a job in spring boot application to run periodically, spring boot provides [@EnableScheduling](https://docs.spring.io/spring/docs/current/javadoc-api/org/springframework/scheduling/annotation/EnableScheduling.html)
and [@Scheduled](https://docs.spring.io/spring/docs/current/javadoc-api/org/springframework/scheduling/annotation/Scheduled.html)
annotations.
@EnableScheduling
is a Spring Context module annotation. It internally imports the [SchedulingConfiguration](https://docs.spring.io/spring/docs/current/javadoc-api/org/springframework/scheduling/annotation/SchedulingConfiguration.html)
via the @Import(SchedulingConfiguration.class)
instruction
@SpringBootApplication
@EnableScheduling
public class SpringBootWebApplication {
}</span>
@Scheduled
This is added to the specific methods you want to schedule. Alot of magic is handled by spring under the hood.
Schedule task at fixed rate
@Scheduled(initialDelay = 1000, fixedRate = 10000)
public void run() {
logger.info("Current time is :: " + Calendar.getInstance().getTime());
}</span>
Schedule task at fixed delay
This will allow the task to run after a fixed delay. In given example, the duration between the end of last execution and the start of next execution is fixed. The task always waits until the previous one is done.
@Scheduled(fixedDelay = 10000)
public void run() {
logger.info("Current time is :: " + Calendar.getInstance().getTime());
}</span>
cron job
cron is a Linux utility which schedules a command or script on your server to run automatically at a specified time and date. A cron job is the scheduled task itself. Cron jobs can be very useful to automate repetitive tasks.
@Scheduled(cron = "0 10 10 10 * ?")
public void run() {
logger.info("Current time is :: " + Calendar.getInstance().getTime());
}</span>
Run The Job Only Once
Most often you will want to schedule the task to run only , there are many fixes around this but for now we can take the simplest .
Dead man’s solution:
You will be dead before it fires again.
@Scheduled(initialDelay = 1000 * 30, fixedDelay=Long._MAX_VALUE_)</span>